Remixはフルスタックフレームワークでバックエンドとフロントエンドがワンソースになってて便利なのですが、じゃあ外部から、たとえばモバイルアプリからRemixプロジェクトのデータのJSONだけ欲しいってなったらどうするの?
今回の記事ではRemix上でAPIエンドポイントを公開する方法を解説します。
前回の記事で使ったRemixプロジェクトを使用します。詳しくは以下を参照してください。
この記事ですること
- APIエンドポイント用のファイルを作成する
- CURLでアクセスしてみる
1. APIエンドポイント用のファイルを作成する
どんなディレクトリ構成にするか迷ったのですが、APIエンドポイントであることを明示的にする形で、 api.posts._indexディレクトリを作成しました。http://localhost:5173/api/postsでアクセスできます。
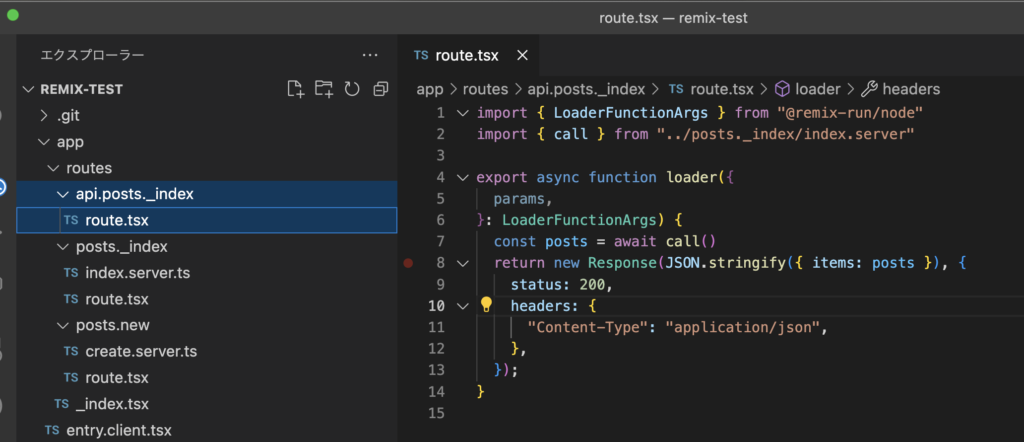
ファイルの中身はこうなっています。
import { LoaderFunctionArgs } from "@remix-run/node"
import { call } from "../posts._index/index.server"
export async function loader({
params,
}: LoaderFunctionArgs) {
const posts = await call()
return new Response(JSON.stringify({ items: posts }), {
status: 200,
headers: {
"Content-Type": "application/json",
},
});
}
UI付きのファイルと見比べてみましょう。
import { json } from "@remix-run/node"
import { Link, useLoaderData } from "@remix-run/react"
import { call } from "./index.server"
import { Post } from "@prisma/client"
export const loader = async () => {
const posts = await call()
return json(posts)
}
export default function PostIndex() {
const posts: Post[] = useLoaderData()
return (
<div>
<h1>Posts</h1>
<ul>
{posts.map((post: Post) => (
<li key={post.id}>
<p>{post.title}: {post.content}</p>
</li>
))}
</ul>
</div>
)
}
APIの方はloaderだけを実装しています。また、APIのloaderではリクエストパラメータはLoaderFunctionArgs、戻り値はResponse型になっています。
2. CURLでアクセスしてみる
CURLでエンドポイントを叩いてみてJSONデータが返ってくるか確認してみましょう!
$ curl http://localhost:5173/api/posts | jq
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 548 0 548 0 0 6441 0 --:--:-- --:--:-- --:--:-- 6765
{
"items": [
{
"id": "abcde",
"title": "This is my new book 1",
"content": "A is the letter before B"
},
{
"id": "clu4262ca0000qb2qq2a0fwo8",
"title": "asdfasdfasd",
"content": "a3aasdfasd"
},
{
"id": "clu426f4d0001qb2qp50p13iq",
"title": "asdfad",
"content": "a433323"
},
{
"id": "clu426r410002qb2qf36ridzj",
"title": "AAAAAA",
"content": "ZZZZZZZZZ"
},
{
"id": "clu427ldy0003qb2q5bnmrwu4",
"title": "CCCCCC",
"content": "EEEEEEEE"
},
{
"id": "clu43ri690000iktkx6y851cl",
"title": "OOOOOOOOO",
"content": "PPPPPPPP"
},
{
"id": "xyz",
"title": "This is my new book 2",
"content": "Z is the last letter"
}
]
}
JSONだけで返ってくることが確認できました。
以上、簡単ではありますが、RemixプロジェクトでAPIエンドポイントを作成する方法の解説でした。
最後までお読みいただきありがとうございました!!